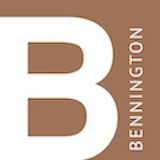
-->
assignments
1. HW0 : getting started
due Fri Oct 23
- resources and tools
- Get a copy of the textbook if you don't already have one.
- Browse through this website - the syllabus and resources particularly.
- Join the course slack channel, #fall20-systems bennington-cs.slack.com. Post a question - any question - on the course slack channel. For more brownie points, answer someone else's question.
- Access the shell terminal and file editor at jupyter.bennington.college. Make sure that you can navigate in the unix shell with shell commands like
ls
and cd
.
- readings
- Get a copy of the textbook if you don't already have one.
- Browse chapter 1 (an overview with some context)
- Start reading chapter 2 (bit representations of data types and C bit operations)
- coding
- Create a
hello.c
file with the classic "Hello World" program in C. Compile and run it.
- Write and run a C program to solve project euler problem 9 : find a,b,c such that \( a+b+c=1000 \) and \( a^2 + b^2 = c^2 \) . Does that code work for \( a+b+c=100000 \) ?
- coming
- The data lab is due in a week. (I'll go over in class what needs to be done.)
- talk to me.
- What is your background? In particular, how comfortable are you with the unix shell and the C programming language?
- What is your internet connectivity like, and what sort of computer and OS are you using?
- How easy or hard was this first assignment?
- Any questions at this point?
2. HW1 : bits and bytes
due Tue Oct 27
- Read chapter 2 in the text, and check out the corresponding CMU lecture notes and/or videos, namely the "bits, bytes, and ints" and "floating point" lecture notes.
- Turn in the following exercises from the text :
- practice problem 2.11 (swap using bit operations) . Explain what's going on.
- practice problem 2.14 (bit and logical operations)
- practice problem 2.23 (byte extraction idioms)
- homework problem 2.60 : implement the "unsigned replace_byte(unsigned x, int i, unsigned char b) function.
- homework problem 2.61 & 2.64 : bit tests without "if" . (And/or do several ways.)
- homework problem 2.76 : implement a robust calloc
- practice problem 2.47 (if we don't do it in class), 5-bit float
- Work on the data lab.
- Coming : we're heading towards machine code and the chapter 3 material in a week ... I would suggest trying to read ahead, looking at the "machine prog" lectures.
3. data lab
due Fri Oct 30
- Do as much as you can of the data lab .
- Submit an explanation of what you did and some of the ideas that these exercises are built on.
- Please also submit the output of running the tests, and what score you got.
- You DO need to try each lab : explore what it is, get it running on jupyter.bennington, and understand the basics.
- You do NOT need to do the whole thing ... but remember that you'll be asked to choose three of the six labs to write-up in more detail .
4. HW2 : machine code 1
due Tue Nov 3
- Continue to explore the material in chapter 3 of the text, learning to understand machine code. The CMU slide and corresponding videos are the "Machine Prog" sessions.
- Work through these practice problems from chapter 3 (all the answers are in the text):
- practice 3.1 : address notation, things like 9(%rax,%rdx)
- practice 3.5 : turning assembly back into C code
- practice 3.6 : understanding LEA (load effective address) instructions
- practice 3.16 : translate conditional jumps into C with GOTO . (If you haven't seen address labels and goto statments in C before, you can see an example here. )
- practice 3.23 : loops in assembly language
- practice 3.32 : understanding the stack
- Explore a running C program with the gdb debugger.
- There are links to gdb tutorials on the resources page.
- You can use a short C program of your choice or my demo.c code version zero.
- Compile with
gcc -Og program.c -o program
(-Og means "optimize for debugging").
- Put in break points at the start of some of the functions. Step through the machine code, and see what happens on the stack and in the registers. Explain what's going for at least one function (swap is the simplest in my demo.c).
- Make a diagram showing where in memory the machine code for the functions, the stack, and the heap is.
- This is an open ended assignment - the point is to get used to gdb ... you'll need it for the bomblab.
- (I will be posting my own version of an exploration of demo.c.)
5. bomb lab
due Fri Nov 6
- Do as much as you can of the bomb lab.
- We're just using the CMU "student self study" one.
- This deadline may spill over into next week if you so choose ... though that means you'll have less time for the attack lab, which is also extremely cool stuff.
- Submit an explanation of what you did and how much of the bomb you were able to disarm.
- You DO need to at least start this lab and play around with it.
- You do NOT have to do the whole thing ... but this has been a popular choice for one to write-up, and is worth diving into in a serious way.
- Questions? Ask.
6. HW3 : machine code 2
due Tue Nov 10
- Finish up with the bomblab if you haven't yet.
- Read and study section 3.10 in the text, on C pointers and stack attacks. Look at the corresponding lecture notes and/or videos, Machine Prog: advanced, Recitation 5: Attack Lab & Stacks, and Recitation 6: C review & C boot camp.
- Write a C program that reads and stores up to 16 strings, using malloc to allocate memory for each string (i.e.
char*
s) and for an array of string pointers (i.e. char**
a). The point here is make sure that you understand (a) what malloc does, and (b) what a C type such as char**
means. Run your program with gdb and/or put in the enough print(pointer) statements to figure out where in memory your code, the heap, and the stack are, and make a diagram showing those memory locations, similar to the one on slide 5 of the "09-machine-advanced" lecture. (This task is related to but not quite the same as these examples, which store input strings in various memory configurations.)
- Explore this buffer overflow code. Explain and diagram what's going wrong, as described in the readme.txt.
7. attack lab
due Fri Nov 13
- Do as much as you can of the attack lab.
- We're still using the CMU "self study" one.
- See my Nov 10 notes for a discussion of how it works and what's to be done.
- Explain what's going on.
8. HW4 : caches
due Tue Nov 17
- Look at the material in chap 6 corresponding to the lecture notes The Memory Hierarchy and Cache Memories.
- We aren't going to spend a lot of time with this, nor are we doing a lab on this material. The cache details get messy, come in different variations, and are changing - this discussion is five years old. But the basic ideas are worth understanding.
- Do practice problem 6.8 , on the ordering of loops. As with all these practice problems, the answers are in the text.
- Try to see that locality effect in loop ordering explicitly by timing some code.
- This matrix_multiply.c code implements one of the matrix multiplication loops shown in Figure 6.44 of our textbook.
- Run it with several different loop order and see if the results are consistent with the text's analysis. Feel free to vary the parameters as you see fit. Things you might want to try: use
double
rather than float
, or bigger N, to change how things fit into the cache. Make REAPEATS smaller to make it run faster.
- If you're doing this on jupyter.bennington,
cat /proc/cpuinfo
can be informative, especially for understanding things like cpu cycles per floating operation, or how much of what is fitting into the caches.
- Do practice problems 6.12, 6.13, and 6.16, which look at the specifics of cache hits, misses, and layout. (The answers are in the textbook.) This summary of cache terminology from the text might be helpful.
9. HW5 : processes
due Fri Nov 20
- Study the material in chapter 8.1 - 8.8, on processes and signals, corresponding to the lecture notes ECF: Exceptions & Processes and ECF: Signals
- Do practice problem 8.4 (number and order of fork outputs)
- Do practice problem 8.6 (print command line and environment strings)
- Do practice problems 8.5 and 8.7 (catch a control-C and print "slept for X of Y seconds")
- Start looking at the shell lab ... there are many pieces to it.
10. shell lab
due Tue Nov 24
- Do as much as you can of the shell lab (tshlab).
- As usual, explain your work.
11. HW6 - virtual memory
due Tue Dec 1
- Read chap 9.1 - 9.5 (virtual memory concepts) and the corresponding material in the CMU "virtual memory" lecture notes and videos.
- Read chap 9.8 (linux process layout, mmap), 9.9 (malloc - stuff for lab), 9.10 (garbage collection), and 9.11 (C memory errors), and check out the corresponding material in the CMU "storage allocation" lectures notes and videos.
- Explain practice problem 9.3 (virtual memory page sizes and parameters)
- Implement practice problem 9.5 (file copy with mmap)
- Explain practice problem 9.6 (details of a heap malloc data)
12. malloc lab
due Fri Dec 4
- Try your hand at the malloc lab.
- I've set up malloclab-bennington.tar which will run correctly on jupyter.bennington.
- Start by reading the writeup .
- As with these other labs, at least do enough to understand the task to be done, the tests, and the provided (naive) partly working code, and explain what you see and what's to be done.
- Your mission is to write your own (malloc, free, realloc) ...
- Here's a short explanation of how to get going.
- The textbook describes and gives some explicit code for a linked-list approach - getting that to work, testing it, and explaining it would be enough for a write-up.
- This lab leaves a lot of room for creativity ...
13. HW7 : networking
due Tue Dec 8
- Study the material in chapter 11, on network client/server coding.
- Suppose you want to run a client/server pair of programs, one on your own computer, and one on jupyter.bennington.college. What is the IP address of those two hosts? And what is an "IP address" in the first place? (Hint: there are many ways to get this information including both command line and cloud tools. But careful - I'm asking for your public IP, not your local network IP. If you don't know what the difference is, google for example "public vs private IP address".) Could you run the server on either one, or would one machine be easier to use as the server? Why or why not? And what are those two IP addresses in hex? (This is a variation on Practice Problem 11.1 in the text.)
- Examine, compile, test, and explain echoclient.c and echoserveri.c , both from chapter 11. (You can find that code, supporting files, a Makefile, and a .tar.gz with all of it in this networks folder, or you can download the pieces from the textbook's code links.) One way to run these is with two jupyter terminals in two different browser windows.
- Optional : starting from echoclient.c and echoserveri.c , build a chat client and server that echos what someone on either end types on the other end. There is working code of mine that does this in the chat/ folder of that networks/ download, so if you want to try this yourself, don't look at mine right away. (Hint: each end needs to do two things ... so fork().)
- Also test, compile, and explain the tiny.c webserver, also in that same networks folder.
- Optional : Learn enough of the HTTP web protocol to fetch a web page, using "telnet jupyter.bennington.college " in one terminal while running "./tiny " in another. (Do you speak HTTP?)
- (I'll do demos of several of these tasks in class. The assignment is essentially just to see if you understand these ideas, and can test that understanding with these bare bones internet clients and servers.)
14. proxy lab ... OPTIONAL
due Fri Dec 11
- OPTIONAL : You don't need to submit anything for this one - feel free to use the time to get your three chosen writeups in shape.
- If you do want to explore it, then use the CMU writeup and their proxylab-handout.tar . Upload the .tar file to a folder on jupyter.bennington, extract the files, and run "make" in both the top folder and in the tiny/ folder.
- The task is to create a proxy which can run between a web client and server, i.e.
web_browser -- proxy -- web_server
.
- There is a lot of internet tech background behind all this - IP addresses, ports, sockets, HTTP protocol, and so on.
- They do NOT give a template for this one ... but the tiny web server and echo client/server in the textbook have all the pieces to create the proxy. From the browser point of view, the proxy acts as a server, and code for that is in tiny.c. From the web_server's point of view, the proxy acts as a client, sending a request, and the echoclient.c program has the code to open a connection and send data.
- Mastering all of this can give you an excellent understanding of the foundation concepts of the internet.
15. three writeups
due Mon Dec 14
- Choose three of the six labs that you would like to count towards your term standing.
- For each write up a brief explanation of what you did, if you haven't done so already. I'm looking for something that another student could use to understand that lab, or part of that lab. Include a bibliography.
- Your overall course evaluation will be based on (a) the number of assignments completed, and (b) those three labs.
- (Note due date change - now Monday after the last day of classes.)
16. Jim's semester feedback
due Fri Dec 18
- A place for Jim to leave feedback for the semester , including the narrative evaluation and a grade if you asked for one.